Understanding PDO in PHP: A Comprehensive Guide
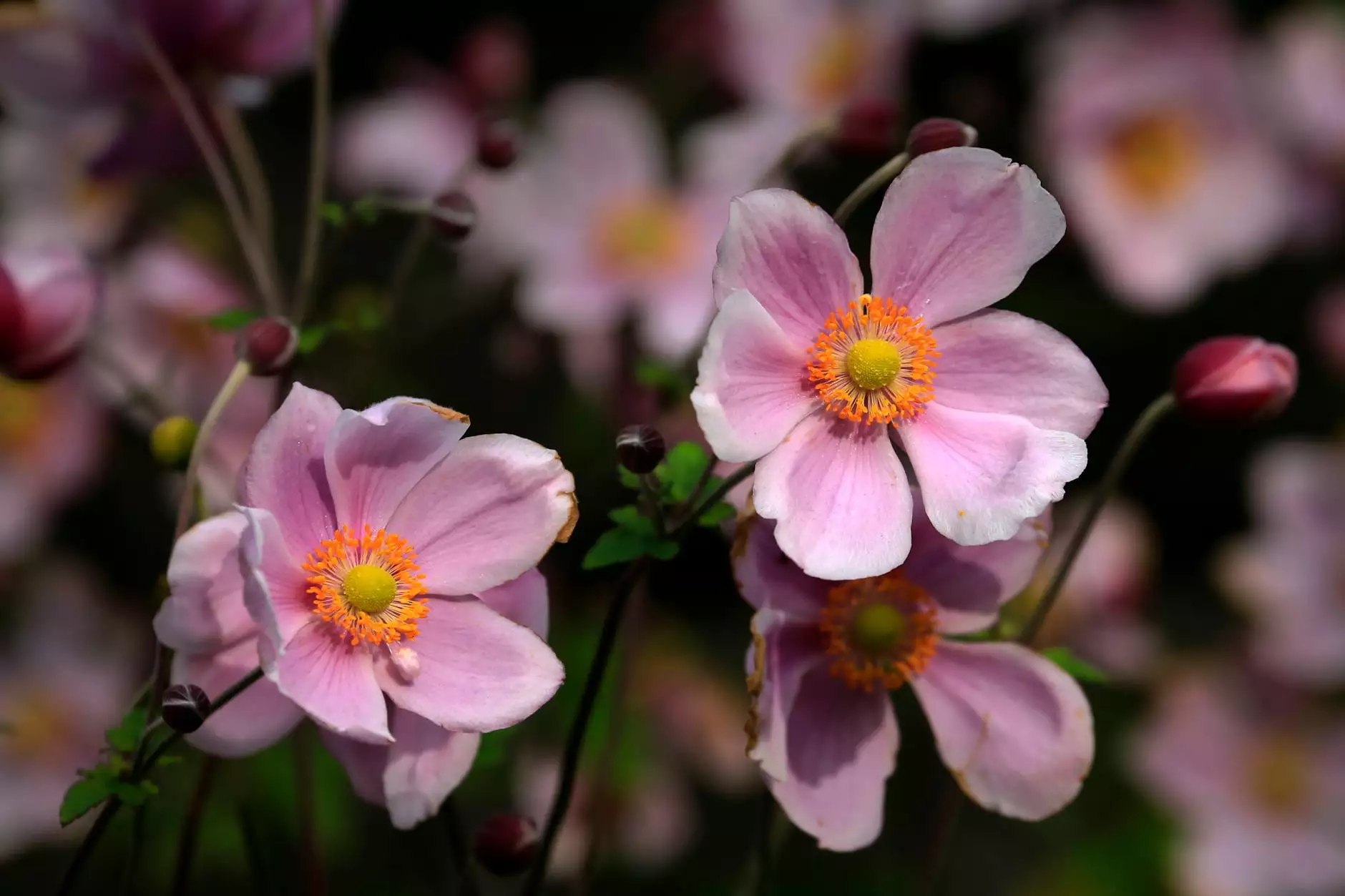
PHP Data Objects (PDO) is an essential database access layer that provides a streamlined and consistent approach to interacting with databases using PHP. By mastering PDO, developers can perform various operations on their databases with enhanced security and efficiency.
What is PDO?
PDO, or PHP Data Objects, offers a versatile way to interface with different types of databases without altering the fundamental code structure. This flexibility allows developers to create applications that can easily switch from one database to another without necessitating significant rewrites of the underlying logic.
The Importance of Database Access Layer
When building data-driven applications, utilizing a robust database access layer like PDO is crucial. Here’s why:
- Security: PDO provides prepared statements, which protect against SQL injection attacks by separating SQL logic from user inputs.
- Flexibility: You can switch databases (MySQL, PostgreSQL, SQLite, etc.) with minimal code alteration.
- Error Handling: PDO allows the developer to define error modes to handle exceptions gracefully.
- Support for Transactions: PDO supports transactions which help in maintaining data integrity.
Getting Started with PDO
To harness the power of PDO, you should first establish a database connection. The following steps showcase how to connect to a MySQL database:
try { $pdo = new PDO('mysql:host=localhost;dbname=your_database', 'username', 'password'); // Set the PDO error mode to exception $pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); echo "Connected successfully"; } catch (PDOException $e) { echo "Connection failed: " . $e->getMessage(); }Understanding Parameter Strings
You may have encountered phrases like pdo pdo :: param_str 0934225077 in programming. This indicates a parameter string used in PDO for executing SQL statements safely. It is crucial for enhancing SQL statements and ensuring security in data transactions.
Using Prepared Statements
Prepared statements are pivotal in PDO. They help in executing SQL queries with parameter markers that are replaced with actual values during execution. Here’s a simple example:
$sql = "SELECT * FROM users WHERE id = :id"; $stmt = $pdo->prepare($sql); $stmt->bindParam(':id', $userId, PDO::PARAM_INT); $userId = 0934225077; // Example user ID $stmt->execute(); $result = $stmt->fetchAll();Benefits of Using PDO
1. Enhanced Security
Using parameterized queries prevents SQL injection, thus safeguarding sensitive information and maintaining the integrity of the data.
2. Consistency Across Multiple Databases
PDO unifies various database interactions to give developers a consistent experience. This allows effortless migration between different database systems, adapting to project requirements.
3. Comprehensive Error Handling
PDO provides robust error handling capabilities. Developers can specify error handling strategies to deal with database errors appropriately and enhance application resilience.
Advanced PDO Usage
Transactions in PDO
Transactions are used when multiple SQL statements need to be executed. They ensure that either all statements are executed successfully or none at all. Here’s how you can implement transactions:
try { $pdo->beginTransaction(); // Start transaction // SQL operations $pdo->exec("INSERT INTO users (name) VALUES ('John Doe')"); $pdo->exec("INSERT INTO users (name) VALUES ('Jane Smith')"); $pdo->commit(); // Commit changes } catch (Exception $e) { $pdo->rollBack(); // Rollback changes if something fails echo "Failed: " . $e->getMessage(); }Fetching Data
Fetching data is straightforward with PDO. You can retrieve data in various formats:
- fetch(): Fetches a single row.
- fetchAll(): Fetches all rows.
- fetchColumn(): Fetches a single column from the next row of the result set.
Best Practices When Using PDO
To maximize the benefits of PDO usage, consider implementing the following best practices:
- Always use prepared statements for user inputs.
- Utilize transactions for operations involving multiple steps.
- Implement detailed error handling to better manage failures.
- Close connections when they are no longer needed.
Conclusion
Understanding and utilizing PDO can significantly improve your PHP application development process. By leveraging its features like prepared statements, error handling, and transactional support, developers can build secure, efficient, and flexible applications. Remember, the strings like pdo pdo :: param_str 0934225077 are more than mere phrases; they encapsulate the power and capability of data handling in modern PHP applications.